Understanding the Power of the const Keyword in C++ Classes
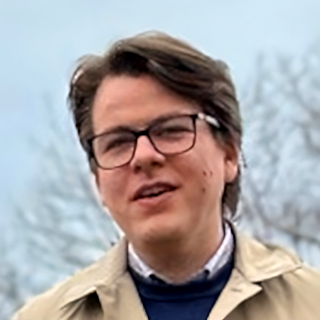
Table of Contents
Overview #
To me the const
keyword is both trivial, but interesting at the same time. Hence with this post I’d like to explore the const
keyword in its usage and how do idealy employ it to harness the benefits of readability, maintainability, and robustness for your code. The const
keyword as you may guess indicates that something in your program is constant and cannot be modified. When applied to classes, the const keyword becomes a valuable tool for ensuring the integrity of your code. So let us explore the significance of the const keyword in C++ classes and its various applications.
Understanding the Basics of const in C++: #
In C++, the const
keyword is used to declare constants and also to specify that a variable, function parameter, or member function does not modify the object it operates on. For example, consider a simple double variable:
const double PI = 3.1416;
In this case, PI is a constant, and its value cannot be changed throughout the program.
Using const with Member Functions #
When const is applied to member functions within a class, it indicates that those functions do not modify the object’s state. This is crucial for ensuring data integrity, especially in large and complex codebases. Here’s how you can declare a const member function:
class MyClass {
public:
void ModifyState() {
// Modify object state
}
void ReadState() const {
// Read object state, cannot modify it
}
};
In this example, the ModifyState()
function can change the object’s state, while the ReadState()
function is marked as const, indicating that it won’t modify the object. If you attempt to modify any member variables within a const member function, the compiler will generate an error. This both adds to the readability, since any user of your class will instantly see that calling this method will not change the class. For a method called ReadState()
this would also be expected, since nothing in its name indicates any change.
Using const Member Functions for Immutable Classes #
Immutable classes are classes whose objects cannot be modified once they are created. By using const member functions, you can achieve immutability, ensuring that objects of the class remain constant throughout their lifetimes. Immutable classes are particularly useful in multithreading scenarios, where data consistency is paramount.
class ImmutableClass {
private:
const int value;
public:
ImmutableClass(int val) : value(val) {}
int GetValue() const {
return value;
}
};
In this example, the member variable value is marked as const, and the GetValue() function is also marked as const, ensuring that the object’s state cannot be modified after initialization.
Best Practices for Using the const
Keyword
#
- Use const for Constants:
Declare constants using const to make the code more readable and understandable. Use const for variables that should not be modified once initialized.
const int MAX_VALUE = 100;
- Use const with Function Parameters:
Use const for function parameters that should not be modified within the function. This helps indicate that the function will not change the input parameters.
void ProcessData(const std::vector<int>& data);
Here the reference of data is passed to the function, but we can expect the contents of data to be unaltered.
- Use const with Member Functions:
Mark member functions that do not modify the object’s state as const. This provides a clear indication of the function’s behavior and allows these functions to be called on const objects.
class MyClass {
public:
int GetData() const;
void PrintData() const;
};
- Use const with Member Variables:
Mark member variables as const if they should not be modified after initialization. This ensures that the data remains constant throughout the object’s lifetime.
class MyClass {
private:
const int constantValue;
public:
MyClass(int value) : constantValue(value) {}
};
- Use const Objects and References:
Use const objects and references to prevent modifications to the underlying data. This is particularly useful when passing objects by reference to functions that should not modify the object.
const MyClass obj;
const MyClass& refObj = obj;
Using const in this manner is beneficial in several scenarios:
- Preventing Modification: It ensures that the state of the object, in this case, obj, cannot be modified inadvertently within a certain scope.
- Passing to Functions: When you pass an object by reference to a function that should not modify the object, you can use const to communicate this intent. For example:
void someFunction(const MyClass& input) {
// The function cannot modify the object referred to by 'input'
// ...
}
// Usage
someFunction(obj); // OK, 'obj' is passed as a const reference
- Be Mindful of const Overloading:
When overloading functions with both const and non-const versions, it’s important to ensure that the behavior of these versions is consistent. The const and non-const overloads should ideally perform similar operations or provide logically equivalent functionality. This is crucial for maintaining a clear and predictable interface for users of your code.
Let’s consider an example to illustrate this point:
#include <iostream>
class MyClass {
private:
int value;
public:
// Non-const member function to modify the internal state
void modifyValue() {
std::cout << "Modifying the value" << std::endl;
// Code to modify the internal state
}
// Const member function to read the internal state
void readValue() const {
std::cout << "Reading the value: " << value << std::endl;
// Code to read the internal state
}
// Non-const version of readValue to emphasize modification capability
void readValue() {
std::cout << "Reading the value (non-const): " << value << std::endl;
// Code to read the internal state
}
};
int main() {
MyClass obj;
// Calling the non-const version
obj.modifyValue();
// Calling the const version
obj.readValue();
const MyClass constObj;
// Calling the const version for a const object
constObj.readValue();
// Error: constObj.modifyValue(); // This would result in a compilation error
return 0;
}
When overloading functions like this, it’s important to maintain a consistent behavior between the const and non-const versions. Users of your class should be able to rely on the const version not modifying the object’s internal state. If the const version of a function modifies the object or if the non-const version performs an operation that is not appropriate for a const object, it can lead to confusion and unexpected behavior.
- Use const Correctly with Pointers:
When using pointers, const can be placed on the pointed data, the pointer itself, or both. Understand the difference between const int* ptr (pointer to constant integer) and int* const ptr (constant pointer to an integer).
const int* ptr; // ptr points to a constant integer
int const* ptr; // Same as above: ptr points to a constant integer
int* const ptr; // ptr is a constant pointer to an integer
const int* const ptr; // ptr is a constant pointer to a constant integer
- Avoid Excessive Use of const_cast:
In C++, const_cast is a type of casting operator that can be used to add or remove the const qualifier from a pointer or reference. The const qualifier is used to indicate that a variable should not be modified. Here’s a brief explanation of the issue you mentioned:
When you use const_cast to cast away the const qualifier from a variable and then modify it, you are essentially telling the compiler, “Trust me, I know what I’m doing, and I won’t actually modify this variable.” However, if the variable was originally declared as const, modifying it through the use of const_cast leads to undefined behavior.
#include <iostream>
int main() {
const int constVar = 42;
// Using const_cast to remove const qualifier
int* nonConstPointer = const_cast<int*>(&constVar);
// Modifying the supposedly non-const variable
*nonConstPointer = 100;
// Undefined behavior here, modifying a const variable
std::cout << "Modified constVar: " << constVar << std::endl;
return 0;
}
In this example, we create a const variable constVar and then use const_cast to obtain a non-const pointer to it. We then modify the value through the non-const pointer. This results in undefined behavior, meaning the program’s behavior is unpredictable and can vary between different compilers or even different runs of the same program.
- Consistency is Key:
Be consistent in your use of const throughout the codebase. Adhere to a consistent naming convention for const variables and functions to enhance code readability.